Few weeks back I designed an application, where I wanted to display Help content through a PDF file rather than through a textbox in a JPanel, because the help content had complex formula stuffs. So I thought of embedding the PDF file with my swing application itself. But it was too tricky to learn in a short span of time. So I figured out two options to display the help content.
- By opening the PDF file using a native PDF Reader in the system, on click of the Help Button in my Java Swing application.
- By opening the PDF file using a PDF reader integrated with my application jar package, on click of the Help Button in my Java Swing application.
One solution would be to load a PDF file from a Web link. Another solution would be to use an installation wizard (See here) for your Java application to place the PDF file in predetermined path, so that you can be sure that you have referred the correct path in your source code.
Method 1 : Using Native PDF Reader
The code for my Help Button looks like this1: private void jButton17ActionPerformed(java.awt.event.ActionEvent evt) {
2: pdfopener pdfo = new pdfopener();
3: pdfo.helpview();
4: }
I am calling the helpview() method of pdfopener class to open the pdf file. Lets look in to the pdfopener class.
1: package metrics.wizard;
2:
3: import java.awt.Desktop;
4: import java.io.File;
5: import javax.swing.JOptionPane;
6:
7: public class pdfopener {
8:
9: public void helpview() {
10: File pdffile = new File("C:/", "sample.pdf");
11:
12: try {
13: Desktop.getDesktop().open(pdffile);
14:
15: } catch (Exception ex) {
16: JOptionPane.showMessageDialog(null, "Unable to load the help file", "File Read Error", JOptionPane.ERROR_MESSAGE);
17: }
18:
19:
20: }
21: }
I’m using File object to handle this. Note in Line 10 that the first argument we pass in to the Constructor of File object is the predetermined path of the PDF file, the second argument is the name of the PDF File. So for example if the path of your PDF file is C:\Program Files\My Swing App\help.pdf , the argument should be
File pdffile = new File("C:/Program Files/My Swing App/", "sample.pdf");
Note that forward slash “/” should be used instead of “\” while referring the address.
Referring by the address “/sample.pdf” will direct you to the root folder irrespective of the Operating System. In case of Windows Operating Systems, “/” will refer to C:\ usually.
You can also notice that I have used try catch statement in Line 16 to handle exceptions. It will do nothing but display user with a presentable Error message.
Method 2 : Using Integrated PDF Reader with your Swing Application
Imagine a scenario where your end user doesn’t have a PDF reader installed in their system. In that case you can supply a PDF reader along with your application to open the PDF file. You need not develop your own PDF reader, thanks to third party tools providers like ICESoft. You can use the ICEpdf Viewer to enable PDF reader capabilities for your Java Application.First you need to download the ICEpdf Viewer tools from here http://www.icesoft.org/downloads/icepdf-downloads.jsf . You could notice that their entire site is made of Java Server Faces, a cool Java technology to build Rich Web pages. Let get back to our topic. Download the binary release of ICEpdf viewer. Unzip the contents. In the unzipped content you can find two jar files icepdf-core.jar and icepdf-viewer.jar in the following path ICEpdf-4.3.3-bin\icepdf\lib\ . These are the files that we need now.
Copy those two files to some location of your preference. I used to copy them in to a folder named Library under My Documents. Now you need to import these files in to your Project.
I’m using Netbeans to develop my application, so I will guide you on how to import the library in to your Netbeans Java Swing project. Right Click on the Project Name in Project Explorer Pane and then Select Properties. Under Categories, select Libraries. Then Click on Add JAR/Folder and select the two jar files that you have extracted. The imported files will look like in below screenshot.
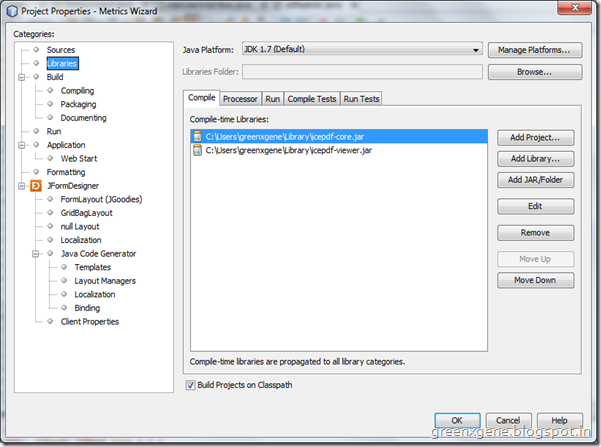
Now getting back to the Project, the code will vary from Method 1 only for the helpview() method. The helpview() source code is given below
1: /*
2: * To change this template, choose Tools | Templates
3: * and open the template in the editor.
4: */
5: package metrics.wizard;
6:
7: import java.net.URISyntaxException;
8: import javax.swing.JFrame;
9: import javax.swing.JPanel;
10: import org.icepdf.ri.common.ComponentKeyBinding;
11: import org.icepdf.ri.common.SwingController;
12: import org.icepdf.ri.common.SwingViewBuilder;
13:
14: /**
15: *
16: * @author greenxgene
17: */
18: public class pdfopener {
19:
20: public void helpview() throws URISyntaxException {
21:
22: String filepath = "C:/sample.pdf";
23:
24:
25:
26: // build a controller
27: SwingController controller = new SwingController();
28: // Build a SwingViewFactory configured with the controller
29: SwingViewBuilder factory = new SwingViewBuilder(controller);
30: JPanel viewerComponentPanel = factory.buildViewerPanel();
31: // add copy keyboard command
32: ComponentKeyBinding.install(controller, viewerComponentPanel);
33:
34: // add interactive mouse link annotation support via callback
35: controller.getDocumentViewController().setAnnotationCallback(
36: new org.icepdf.ri.common.MyAnnotationCallback(
37: controller.getDocumentViewController()));
38:
39: // Use the factory to build a JPanel that is pre-configured
40: //with a complete, active Viewer UI.
41:
42: // Create a JFrame to display the panel in
43: JFrame window = new JFrame("Metrics Wizard Help");
44:
45: window.getContentPane().add(viewerComponentPanel);
46: window.pack();
47:
48: window.setVisible(true);
49:
50: // Open a PDF document to view
51: controller.openDocument(filepath);
52: }
53: }
You can notice that we are declaring a String variable to hold the file path in Line 22. Unlike in method the the file path and file name can be included in the same variable. the rest of the codes are for building the pdf viewer from the library. The variable with the file name and path is passed on to the viewer at Line 51.
Also note that the declaration at Line 43 provides the title for the PDF File.
The code for Help button that launches the PDF file will be same as in Method 1, so once you launch the PDF File through ICEpdf Viewer it will look like in the below screenshot.
Hope this will be useful for building your application with PDF capabilities. Please feel free to post your opinions or queries.
Comments
Post a Comment